What is Angular Pipe?
A pipe takes in data as input and transforms it into an output. The pipe’s purpose is to allow the transformation of an existing value and reusability!
Pipes are simple functions to use in template expressions to accept an input value and return a transformed value. Pipes are useful because you can use them throughout your application, while only declaring each pipe once. For example, you would use a pipe to show a date as April 15, 1988 rather than the raw string format.
We can transform and reuse any data on the template without writing code in the component. Moreover, we can do this with high performance and immutable with Pipes. In addition, Pipes enable data to be processed while a workflow is in progress.
How to Use Angular Pipe?

Angular has ready-made pipes that come with rxjs.
Pipes
1. DatePipe

Angular date pipe is used to format dates in angular based on given date formats, timezone and country locale information.
Using the date channel we can convert a date object, a number (from UTC to milliseconds) or an ISO date string to given predefined angular date formats or custom angular date formats.
Angular date pipe accpets three parameters
- Format
- Timezone
- Locale
I have created a date pipe component in my Angular project and added current datetime values in different formats like milliseconds,date object,datetime string,ISO datetime string.
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-datepipe', templateUrl: './datepipe.component.html', styleUrls: ['./datepipe.component.scss'] }) export class DatepipeComponent implements OnInit { todayNumber: number = Date.now(); todayDate : Date = new Date(); todayString : string = new Date().toDateString(); todayISOString : string = new Date().toISOString(); constructor() { } ngOnInit() { } }
Now in my component I am displaying them using angular date pipe as shown below.
<p>DateTime as Milliseconds : {{todayNumber}} datepipe:{{todayNumber | date}}</p> <p>DateTime as object : {{todayDate}} datepipe:{{todayDate | date}}</p> <p>DateTime as string : {{todayString}} datepipe:{{todayString | date}}</p> <p>DateTime as iso string : {{todayISOString}} datepipe:{{todayISOString | date}}</p> Output: DateTime as Milliseconds : 1560617468681 datepipe:Jun 15, 2019 DateTime as object : Sat Jun 15 2019 22:21:08 GMT+0530 (India Standard Time) datepipe:Jun 15, 2019 DateTime as string : Sat Jun 15 2019 datepipe:Jun 15, 2019 DateTime as iso string : 2019-06-15T16:51:08.681Z datepipe:Jun 15, 2019
All types of datetime values displays the date in ‘MMM d, y’ format which is default Angular date format ‘mediumDate’
To change the datetime format in angular we have to pass date time format parameter to the angular pipe as shown below
{{ date_value | date :'short'}} // 6/15/19, 5:24 PM
The format ‘short’ is one of the predefined date formats in angular which converts our date value to ’M/d/yy, h:mm a’ format.
2. UpperCasePipe
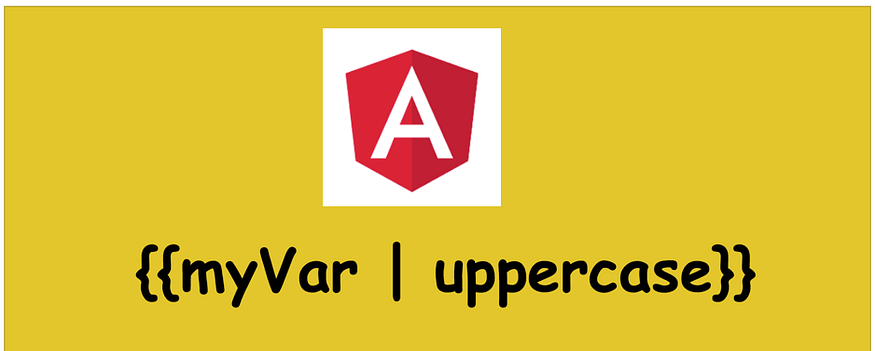
To convert string or text to uppercase in Angular we can use Angular UpperCase Pipe.
Pass input string to the uppercase pipe as shown below.
<p>{{'uppercasepipe convert string to uppercase in angular' | uppercase}}</p> <!-- Output --> <!--UPPERCASEPIPE CONVERT STRING TO UPPERCASE IN ANGULAR-->
Angular UpperCase Pipe is one of the built in pipes which converts text to uppercase.
UpperCase Pipe Usage & Syntax
The syntax is very similar to other built in pipes, we need to pass input string to uppercase pipe without any parameters
{{ string | uppercase }}
UpperCase Pipe Examples
We go through the different types of strings and convert them to uppercase using Angular UpperCasePipe.
We will create a component called uppercase component in our Angular project, will convert different kind of inputs to uppercase.
<p>{{'angular uppercase' | uppercase}}</p> <!-- output is "ANGULAR UPPERCASE" --> <!-- Ultra numeric string to uppercase --> <p>{{'angular version 9' | uppercase}}</p> <!-- output is "ANGULAR VERSION 9" -->
Error: InvalidPipeArgument for pipe ‘UpperCase Pipe’
Angular uppercase pipe accepts only string types. If you pass other types like number or object etc. you will get Error: InvalidPipeArgument for pipe 'UpperCasePipe'
error.
{{ 1 | uppercase}}
The above code won’t compile at all and angular cli returns following error
error TS2345: Argument of type '1' is not assignable to parameter of type 'string'.
We will test uppercase pipe with other type variables.
export class UpperCasePipeComponent implements OnInit {
id : number; products : Product[];constructor() { this.id = 123; this.products = [ {id:1,name:"product1"}, {id:2,name:"product2"} ]; }} interface Product{ id: number; name : string; }
And now angular cli will return error TS2345: Argument of type 'number' is not assignable to parameter of type 'string'.
error when we try to execute below code.
{{ id | uppercase}}
Same with the type product
{{ products | uppercase}}
error TS2345: Argument of type 'Product[]' is not assignable to parameter of type 'string'.
Now we will test uppercase pipe with variable of type any
.
uppercasevariable: any;
constructor(){ this.uppercasevariable = 1; } {{ uppercasevariable | uppercase}}
And now there will be no compile time errors and at run time we will get following error.
Error: InvalidPipeArgument: ‘1’ for pipe ‘UpperCasePipe’
and in addition to that you will get another error
Error: ASSERTION ERROR: Stored value should never be NO_CHANGE. [Expected=> [object Object] !== [object Object] <=Actual]
If you pass object to uppercase pipe you will get following error. I mean when I assign a object to variable type of any
.
Error: InvalidPipeArgument: ‘[object Object]’ for pipe ‘UpperCasePipe’
If you assign string to any
type variable uppercase pipe will work as expected.
3. LowerCasePipe
To convert a string to small letters in template HTML file, pass the input string to the lowercase pipe using pipe operator as shown below.
<p>{{'LOWERCASE pipe convert string to small letters in ANGULAR' | lowercase}}</p> <!-- Output --> <!--lowercase pipe convert string to small letters in angular-->
It’s one of the built-in pipes in Angular and very handy pipe similar to uppercase pipe.
Lowercase Pipe Usage & Syntax
The syntax is very similar to other built in pipes, we need to pass input text to the lowercase pipe without any parameters.
{{ text | lowercase }}
LowerCase Pipe Examples
Now We will go through the different types of strings and convert them to small case letters using Angular LowerCase Pipe.
Let’s create a component called lowercasepipe in our Angular project.
ng generate component lowercasepipe
// CREATE src/app/lowercasepipe/lowercasepipe.component.html (28 bytes) CREATE src/app/lowercasepipe/lowercasepipe.component.spec.ts (677 bytes) CREATE src/app/lowercasepipe/lowercasepipe.component.ts (304 bytes) CREATE src/app/lowercasepipe/lowercasepipe.component.scss (0 bytes) UPDATE src/app/app.module.ts (3991 bytes)import { Component, OnInit } from '@angular/core';@Component({ selector: 'app-lowercasepipe', templateUrl: './lowercasepipe.component.html', styleUrls: ['./lowercasepipe.component.scss'] }) export class LowercasepipeComponent implements OnInit { constructor() { } ngOnInit(): void { }}
Convering ultra numerica string to lowercase
<p>{{'ANGULAR LOWERCASE' | lowercase}}</p> <!-- output is "angular lowercase" -->
<!-- Ultra numeric string to lowercase --> <p>{{'ANGULAR version 11' | lowercase}}</p> <!-- output is "angular version 11" -->
Error: InvalidPipeArgument for pipe ‘LowerCase Pipe’
Angular lowercase pipe accepts only string types.
If we pass other types like number or object etc. we will get
Error: InvalidPipeArgument for pipe 'LowerCasePipe'
error.
{{ 1 | lowercase}}
The above code won’t compile and angular cli returns following error
TS2345: Argument of type '1' is not assignable to parameter of type 'string'.
We will test lowercase pipe with other type variables.
export class LowercasepipeComponent implements OnInit {
id : number; products : Product[];constructor() { this.id = 123; this.products = [ {id:1,name:"product1"}, {id:2,name:"product2"} ]; }} interface Product{ id: number; name : string; }
In file lowercasepipe.component.html
file
{{ id | lowercase}}
And now angular cli will return error TS2345: Argument of type 'number' is not assignable to parameter of type 'string'.
error when we try to compile below code.
Same with the type product
{{ products | lowercase}}
error TS2345: Argument of type 'Product[]' is not assignable to parameter of type 'string'.
Now we will test lowercase pipe with variable of type any
.
lowercasevariable: any;
constructor(){ this.lowercasevariable = 1; } {{ lowercasevariable | lowercase}}
And now there will be no compile time errors and at run time we will get following error.
Error: Invalid Pipe Argument: ‘1’ for pipe ‘Lower Case Pipe’
If you pass object to lowercase pipe you will get following error.
I mean when we assign a object to variable type of any
.
Error: Invalid Pipe Argument: ‘[object Object]’ for pipe ‘LowerCasePipe’
If you assign string to any
type variable lowercase pipe will work as expected.
3. Currency Pipe
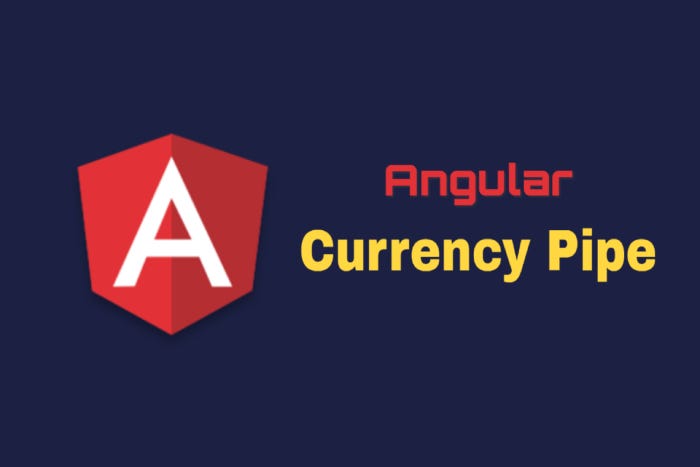
Angular Currency Pipe is one of the built in pipe in Angular used to format currency value according to given country code,currency,decimal,locale information.
How to use Angular Currency Pipe
Angular Currency Pipe takes currency value as input and currency Code, display, digits Info and locale as parameters as shown below
{{currency_value|currency[:currencyCode[:display[:digitsInfo[:locale]]]]}}
Parameter Description currency Code ISO 4217 currency code.Of type string default value undefined, Optional display We can display Currency ‘symbol’ or ‘code’ or ‘symbol-narrow’ or our own string.Of type string, Optional Default value is ‘symbol’ digits Info Represents Decimal representation of currency value.Of type string default value undefined, Optional locale represents locale format rules. Default value is our project locale if set or undefined.Optional
Angular Currency Pipe example
We will go through few examples to understand Angular Currency Pipe.For example we have Currency of Value 100.23.
<!--output '$100.23'--> <p>{{Value | currency}}</p>
<!--output '₹100.23'--> <p>{{Value | currency:'INR'}}</p>
If you are not passing any parameters then it displays the default currency as USD i.e., dollars.
We can change this default currency as explained below.
If we are passing currency code to the angular currency pipe.It will display the corresponding default currency symbol.
I am passing currency code as “INR” and Rupee symbol will be displayed.
Angular Currency Pipe without symbol
If you want to display your own name instead of default currency symbol you have to pass display parameter.
The display parameter can be “code” (currency code will be displayed) or “symbol” or “symbol-narrow” or any other custom value.
<!--output 'INR100.23'--> <p>{{Value | currency:'INR':'code'}}</p>
<!--output 'CA$100.23'--> <p>{{Value | currency:'CAD':'symbol'}}</p><!--output '$100.23'--> <p>{{Value |currency:'CAD':'symbol-narrow'}}</p><!--output '₹100.23'--> <p>{{Value |currency:'INR':'symbol-narrow'}}</p><!--output 'Indian Rupee100.23'--> <p>{{Value | currency:'INR':'Indian Rupee'}}</p>
Few countries like Canada have two currency codes like symbol CA$ and symbol-narrow $. If the country locale does not have symbol-narrow, default symbol will be displayed.
Indian rupee does not have symbol-narrow so the default rupee symbol displayed.
If you want to display your own custom name as symbol you can pass as a parame ter as shown in last example.I am passing ‘Indian Rupee’ as display parameter.
Angular Currency Pipe No Decimal
If you want to customize the decimal points of currency value. You need to pass digit Info parameter.
The syntax of the digit Info parameter is “numberOfInteger:minimumFractions-maxFractions”.
<!--output '₹0,100.35'--> <p>{{Value |currency:'INR':'symbol':'4.2-2'}}</p>
<!--output '₹100.2'--> <p>{{Value |currency:'INR':'symbol':'3.1-1'}}</p>
The first example displayed as “₹0,100.35” because we are telling the currency pipe to display at least 4 digits. Remaining one digit adjusted with 0.
And after the decimal point minimum 2 fractions and maximum 2 fractions are displayed.
In the second example I am passing fractions as ‘1–1’. So it displayed as “₹100.2”.
Angular Currency Pipe by default displays two decimal points irrespective of currency type. If currency is 100.
<!--₹100.00--> <p>{{IntegerValue |currency:'INR':'symbol'}}</p>
To remove decimal points from the Angular currency pipe, we need to pass digit Info parameter fractions as zero.
₹100 <p>{{IntegerValue |currency:'INR':'symbol':'3.0'}}</p>
Few country currencies does not support cent values in that case decimal points are truncated. For example Chilean peso Currency CLP does not support cents. So if the currency value is 100.23. It is displayed as 100.
You can see the below table for list of such countries.
CLP100 <p>{{Value | currency:'CLP':'symbol'}}</p>
Angular Currency Pipe example with locale
We can pass local as parameter to Angular currency Pipe as shown below.
<p>{{Value | currency:'CAD':'symbol':'4.2-2':'fr'}}</p>
But the above code returns the error in console saying Missing locale data for the locale “fr”.
We are passing french locale information as “fr”. But in our application we don’t have locale information for french.
We need to register the locale information.
import { registerLocaleData } from '@angular/common'; import localeFr from '@angular/common/locales/fr'; registerLocaleData(localeFr, 'fr');
Follow the below steps to use Angular Currency Pipe with locale.
- Import the register Locale Data from @angular/common
- Import locale Information from @angular/common/locales/fr.
- And Finally register the information using register Locale Data method.
<!--output '0 100,23 $CA'--> <p>{{Value | currency:'CAD':'symbol':'4.2-2':'fr'}}</p>
<!--output '100,23 $CA'--> <p>{{Value | currency:'CAD':'symbol':'3.2-2':'fr'}}</p>
Display Currency symbol to the right using Angular Currency Pipe
A lot of europe countries use the currency symbol at the right side of currency value (France, Germany, Spain, Italy countries).
If you pass the locale information the symbol will be automatically displayed at the right side of value as shown in above french locale.
But the remaining currencies without locale still displays currency symbol to the left only.
import {NgModule, LOCALE_ID} from '@angular/core';
@NgModule({ providers: [{ provide: LOCALE_ID, useValue: 'fr' // 'de' for Germany, 'fr' for France ... }] })
Import LOCALE_ID from ‘@angular/core’ and pass it to the provides as shown above.
But this will change the locale across the application. So this approach is acceptable only if your site is using single locale information.
And the decision to show the currency symbol to the left or right should be based upon locale information only.
So its better to pass locale information to the Angular Currency Pipe whenever required.
Creating Custom Angular Currency Pipe
What if we want to change default parameters of Angular Currency Pipe like default currency code,default currency symbol, default decimal locations and default locale?
For example we have a fixed currency format across the application as shown below.
{{ Value | currency:'EUR':'symbol':'3.2-2':'fr' }} 100,23 €
Each time I do not want to pass the other parameters and set the above parameters as default parameters so that the below code should display the required format.
{{ Value | currency }} 100,23 €
There is no way we can set the default parameters in Angular Currency Pipe. But we can define our own custom Angular currency Pipe to do the same.
Create a new file called custom.currencypipe.ts file and add the below code to it. You can change the paramters as per your requirements.
import { Pipe, PipeTransform } from '@angular/core'; import { formatCurrency, getCurrencySymbol } from '@angular/common'; @Pipe({ name: 'mycurrency', }) export class MycurrencyPipe implements PipeTransform { transform( value: number, currencyCode: string = 'EUR', display: | 'code' | 'symbol' | 'symbol-narrow' | string | boolean = 'symbol', digitsInfo: string = '3.2-2', locale: string = 'fr', ): string | null { return formatCurrency( value, locale, getCurrencySymbol(currencyCode, 'wide'), currencyCode, digitsInfo, ); } }
And after declaring _MyCurrencyPipe._ Import it in app.module.ts file and add it in declaration array of _app.module.ts. _As shown below.
import {MycurrencyPipe} from './custom.currencypipe';
@NgModule({ declarations: [ MycurrencyPipe ]);
Now i can use my custom currency pipe as show below.
{{ Value | mycurrency }} 100,23 €
5. Decimal Pipe

Angular Decimal Pipe is one of the bulit in pipe in Angular used to format decimal numbers according to the given decimal digits info and locale information.
How to use Angular Decimal Pipe
Angular decimal pipe acceps two parameters
- decimal digit info
- locale
{{ numeric_value | number [ : digitsInfo [ : locale ] ] }}
Angular Decimal Pipe Examples
Now we will go through few angular decimal pipe examples to understand it further.
I created a decimal pipe component in my Angular project and added decimal number variable called decimal_value
.
import { Component, OnInit } from '@angular/core';
@Component({ selector: 'app-decimalpipe', templateUrl: './decimalpipe.component.html', styleUrls: ['./decimalpipe.component.scss'] }) export class DecimalpipeComponent implements OnInit { decimal_value: number = 5.123456789; constructor() { } ngOnInit() { }}
First we will use decimal pipe without parameters.
No Parameters: {{ decimal_value | number }}
// No Parameters: 5.123
As explained above if none of the decimal digit info is given, then decimal pipe will take default options, 1 interger value before decimal point and 3 fraction digits after decimal point.
So the above code is same as
{{ decimal_value | number:'1.0-3' }}
Now we will pass digit informaion paramter to decimal pipe and see how it works
Digit Info Parameter (3.1-5): {{ decimal_value | number:'3.1-5' }}
In the above code we are instructing decimal pipe to show atleast 3 integer values before decimal points and minimum 1 fraction digit, maximum 5 fraction digits.
Digit Info Parameter (3.1-5): 005.12346
As our decimal value contains only single digit before decimal point two extra zeroes are padded two satisfy given digit info.
Angular decimal pipe example with country locale
To display numbers according to country locale format rules, We have to pass country locale code as a second parameter to angular decimal pipe.
To display number in french locale in Angular use locale code ‘fr’ as parameter as shown below.
Decimal pipe with locale france {{decimal_value | number:'4.5-5':'fr'}}
If you execute above code you will get following error in console.
InvalidPipeArgument: 'Missing locale data for the locale "fr".' for pipe 'DecimalPipe'
Because In our application we dont have locale information for ‘fr’
To add the country locale information refer Angular currency pipe article.
After adding locale information you can see the number has been displayed according to france locale rules.
Decimal pipe with locale france 0 005,12346
Angular Decimal Pipe rounding
By default Angular decimal pipe rounds off the number to the nearest value using Arithmetic rounding method.
numeric_value : number = -2.15;
{{ numeric_value | number:'1.1-1'}} // -2.2numeric_value : number = -2.11;{{ numeric_value | number:'1.1-1'}} // -2.1
This is different from JavaScript’s Math.round() function.
For example if the number is -2.5
{{value | number : '1.0-0'}} // -3.
Math.round(value) //-2
If the number is -2.1
{{value | number : '1.0-0'}} //-2
// rounding_value : number = 2.23; {{ rounding_value | number : '1.1-1'}} 2.2// rounding_value : number = 2.25; {{ rounding_value | number : '1.1-1'}} 2.3
Rounding Angular number to 2 decimal points
In most of the real world cases, we will round off numbers to 2 decimal places.
So using decimal pipe we can easily format numbers in Angular upto 2 decimal points by passing digit info as x.2-2
(x is number of digits before decimal points)
// rounding_value : number = 2.25234234234; {{ rounding_value | number : '1.2-2'}}
// 2.25
Angular Decimal Pipe with No decimal points
If you want to remove decimal points and rounding to nearest integet values we can pass digit info as x.0-0
. (x is minimum number of digits before decimal)
//decimal_value: number = 5.123456789;
Without decimal {{decimal_value | number:'1.0-0'}} // 5//decimal_value: number = 5.523456789;{{decimal_value | number:'1.0-0'}} //6
How to use decimal pipe in Components in Angular
As explained in How To Use Angular Pipes in Components & Service ts files article.
Follow the below steps to use decimal pipe in components
- Import DecimalPipe from @angular\common in app.module.ts file
- Add the DecimalPipe to provider array.
- Inject the DecimalPipe in component constructor file using dependency injection.
- And finally format the numbers using decimal pipe transform method as shown below.
export class DecimalpipeComponent implements OnInit {
decimal_value: number = 5.123456789; constructor(private _decimalPipe: DecimalPipe) { var decimal_formatted = this._decimalPipe.transform(this.decimal_value,"1.2-2") console.log(decimal_formatted); } }// In console 5.12
format Number() method in Angular
The above approach requires us to inject decimal pipe in constructor.
Instead of that we can use formatNumber()
method to format numbers in component and service files similarly as decimal pipe.
We can import formatNumber()
from @angular/common
and use it in component file.
import { Component, OnInit, Inject, LOCALE_ID } from '@angular/core'; import { DecimalPipe,formatNumber } from '@angular/common';
export class DecimalpipeComponent implements OnInit { decimal_value: number = 5.123456789; constructor(@Inject(LOCALE_ID) private locale: string) { var formattedNumber= formatNumber(this.decimal_value,this.locale,'1.2-2'); console.log(formattedNumber); }// In console 5.12

Removing Comma from number angular decimal pipe
Angular decimal pipe adds commas to the number according to counry locale rules.
//decimal_value: number = 55234.342342;
{{decimal_value | number:'1.2-2'}} 55,234.34
And there is no direct way to remove those commas from number using decimal pipe.
For that purpose we need to create our own custom pipe which removes commas from formatted number.
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({ name: 'removeComma' }) export class RemoveCommaPipe implements PipeTransform { transform(value: string): string { if (value !== undefined && value !== null) { return value.replace(/,/g, ""); } else { return ""; } } }
And we need apply this removeComma
Pipe after using decimal pipe.
{{decimal_value | number:'1.2-2' | removeComma}}
//55234.34
Error: InvalidPipeArgument: ‘not a valid digit info’ for pipe ‘DecimalPipe’
We need to pass digit information parameter in following format “X.X-X”
{minimumIntegerDigits}.{minimumFractionDigits}-{maximumFractionDigits}
If you pass the parameter in wrong format for instance as shown below
{{decimal_value | number:'1-2.2'}}
You will get following error in console.
Error: InvalidPipeArgument: ‘1–2.2 is not a valid digit info’ for pipe ‘DecimalPipe’
6.Percent Pipe

Transforms a number to a percentage string, formatted according to locale rules that determine group sizing and separator, decimal-point character, and other locale-specific configurations.
Angular PercentPipe
is an angular Pipe
API that formats a number as a percentage according to locale rules. It belongs to CommonModule. Find the syntax.
number_expression | percent[:digitInfo]
Find the description.
number_expression: An angular expression that will give output a number.
percent : A pipe keyword that is used with pipe operator and it converts number into percent.
digitInfo: It defines a percentage format. We have described the use of digitInfo in DecimalPipe
section. It is used with following syntax.
{minIntegerDigits}.{minFractionDigits}-{maxFractionDigits}
Now find some sample examples.
1. Using default format:
minIntegerDigits = 1
minFractionDigits = 0
maxFractionDigits = 3
Now find a number that will be changed into percentage.
num1 = 2.5;
Now use Percent Pipe
{{num1 | percent}}
Find the output.
250%
2. Use format ‘2.2–5’
minIntegerDigits = 2
minFractionDigits = 2
maxFractionDigits = 5
Now find the number that will be changed into percentage.
num1 = 2.5;
Now use Percent Pipe.
{{num1 | percent:'2.2-5'}}
Find the output.
250.00%
We will observe that there is two digits in fraction part. This is because minimum fraction digits required is 2.
Now find the component used in our example.
percentpipe.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'percent-app', template: ` <h3>Percent Pipe</h3> <div> <p> {{num1 | percent}} </p> <p> {{num1 | percent:'2.2-5'}} </p> <p> {{num2 | percent:'1.2-5'}} </p> <p> {{num1 * num2 | percent:'1.2-3'}} </p> </div> ` }) export class PercentPipeComponent { num1: number = 2.5; num2: number = 0.5; }
Find the output.
Percent Pipe 250% 250.00% 50.00% 125.00%
Conclusion
In this article, we learned the structure of angular pipes and how to use them. Thank you. See you in the next article…
- 登录 发表评论