As a frontend developer, coding forms is a habit. We put forms everywhere to add user data through an application, and some parts of them are often repeated or reused within same organisation’s apps and libs.
Goal
The goal of this article is to show a clean way to code a reusable form section, as it could be shared by the design system of your organisation for example.
How to split a form into reusable parts?
- Isolate the form section to be reusable
- Transform it into component
- Make this form section robust using Validation and Unit tests
- Make Angular consider this component as a standard form input using
ControlValueAccessor
interface
In order to keep this article synthetic, we will only focus on the 4th part here!
Why this article ?
The Angular documentation about ControlValueAccessor is pretty weak and the most of dedicated articles only explain the basic usage of it : creating a custom input recognized by Angular forms.
Here we want to split a part of a form composed of multiple inputs to make it standalone and reusable as if it were a single input.
This approach is quite well explained at the end of this article but does not show a running full code example.
Prerequisites
- Angular basics
- Reactive forms
- Basic usage of ControlValueAccessor:
Angular Custom Form Controls - Complete Guide
The Angular Forms and ReactiveForms modules come with a series of built-in directives that make it very simple to bind…
Practice
See the result
If you already cannot wait for the running example, you can have a look here. Or study only the code.
Context
In the below example we will simulate a CV creation platform with:
- a CV form to add a new CV
- a list of already added CVs
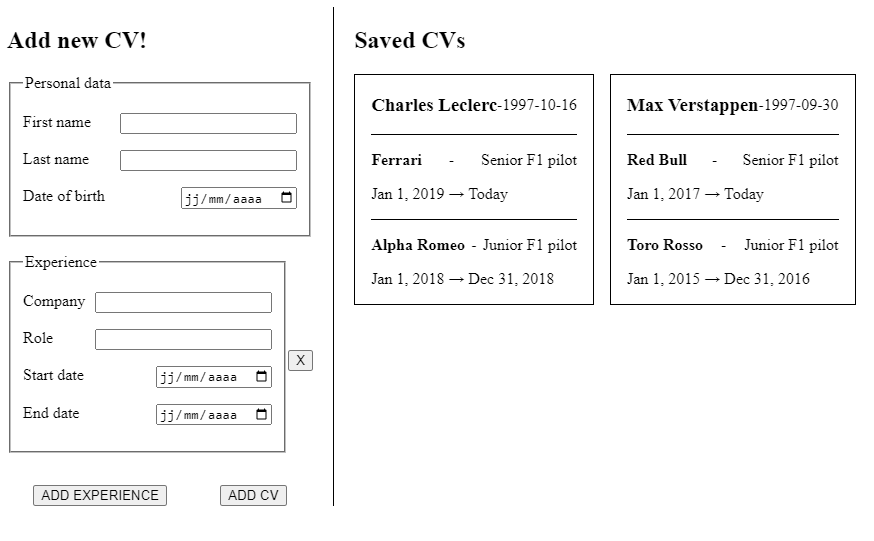
A CV will be constituted of some applicant’s personal data and a list of work experiences. Each experience will have its own related data.
export interface Cv { firstName: string; lastName: string; birthDate: Date; experiences: Experience[]; } export interface Experience { company: string; role: string; startDate: Date; endDate: Date | null; }
Initial CV form
Here is the code of the above form:
<form [formGroup]="cvGroup" (submit)="addCv($event)"> <fieldset> <legend>Personal data</legend> <p> <label for="firstName">First name</label> <input type="text" id="firstName" formControlName="firstName" /> </p> <p> <label for="lastName">Last name</label> <input type="text" id="lastName" formControlName="lastName" /> </p> <p> <label for="birthDate">Date of birth</label> <input type="date" id="birthDate" formControlName="birthDate" /> </p> </fieldset> <ul> <!-- TODO: Add a dynamic list of work experience forms --> </ul> <div class="cv-form-actions"> <button type="button" (click)="addExperience()">ADD EXPERIENCE</button> <button type="submit">ADD CV</button> </div> </form>
We can observe a first fieldset
to collect applicant personal data with standard inputs firstName
, lastName
and birthDate
, and then we would like to implement a dynamic list of forms, where each form would collect the details of a job experienced by the applicant.
Experience form
To achieve this goal, we create a form dedicated to a work experience:
<fieldset [formGroup]="experienceGroup"> <legend>Experience</legend> <p> <label for="company">Company</label> <input type="text" id="company" formControlName="company" (blur)="onTouched()" /> </p> <p> <label for="role">Role</label> <input type="text" id="role" formControlName="role" (blur)="onTouched()" /> </p> <p> <label for="startDate">Start date</label> <input type="date" id="startDate" formControlName="startDate" (blur)="onTouched()" /> </p> <p> <label for="endDate">End date</label> <input type="date" id="endDate" formControlName="endDate" (blur)="onTouched()" /> </p> </fieldset>
The experience form is composed of afieldset
with some related standard inputs as company
, role
, startDate
and endDate
.
Now we want this component to generate an Experience
instance as we declared it before.
1. First we need a FormGroup
representing the work experience form:
experienceGroup = new FormGroup({ company: new FormControl('', { nonNullable: true, validators: Validators.required, }), role: new FormControl('', { nonNullable: true, validators: Validators.required, }), startDate: new FormControl(new Date(), { nonNullable: true, validators: Validators.required, }), endDate: new FormControl(null), });
2. Then we want to trigger a form value change when the experience form is valid:
private _detroyed = new Subject<void>(); ngOnInit(): void { this.experienceGroup.valueChanges .pipe( filter( (experience: Partial<Experience>) => this.experienceGroup.valid && !!experience.company?.trim() && !!experience.role?.trim() && !!experience.startDate ), takeUntil(this._detroyed) ) .subscribe((experience: Experience) => this._onChange(experience)); } ngOnDestroy(): void { this._detroyed.next(); this._detroyed.complete(); } private _onChange = (experience: Experience) => {};
Note that we trigger experience value change only when company
, role
and startDate
are correctly filled.
3. And finally we add the ControlValueAccessor
implementation to make Angular being able to recognize our component as a FormControl
:
onTouched = () => {}; registerOnChange(fn: (experience: Experience) => void): void { this._onChange = fn; } registerOnTouched(fn: () => void): void { this.onTouched = fn; } writeValue(experience: Experience | undefined | null): void { this.experienceGroup.setValue(experience ?? EXPERIENCE_DEFAULT, { emitEvent: false, }); } setDisabledState(isDisabled: boolean): void { isDisabled ? this.experienceGroup.disable() : this.experienceGroup.enable(); } private _onChange = (experience: Experience) => {};
All gathered results in:
export const EXPERIENCE_DEFAULT: Experience = { company: '', role: '', startDate: new Date(), endDate: null, }; @Component({ selector: 'experience-form', standalone: true, imports: [CommonModule, ReactiveFormsModule], templateUrl: './experience-form.component.html', styleUrls: ['./experience-form.component.scss'], providers: [ { provide: NG_VALUE_ACCESSOR, multi: true, useExisting: forwardRef(() => ExperienceFormComponent), }, ], }) export class ExperienceFormComponent implements ControlValueAccessor, OnInit, OnDestroy, Validator { experienceGroup = new FormGroup({ company: new FormControl(EXPERIENCE_DEFAULT.company, { nonNullable: true, validators: Validators.required, }), role: new FormControl(EXPERIENCE_DEFAULT.role, { nonNullable: true, validators: Validators.required, }), startDate: new FormControl(EXPERIENCE_DEFAULT.startDate, { nonNullable: true, validators: Validators.required, }), endDate: new FormControl(EXPERIENCE_DEFAULT.endDate), }); disabled = false; private _detroyed = new Subject<void>(); ngOnInit(): void { this.experienceGroup.valueChanges .pipe( filter( (experience: Partial<Experience>) => this.experienceGroup.valid && !!experience.company?.trim() && !!experience.role?.trim() && !!experience.startDate ), takeUntil(this._detroyed) ) .subscribe((experience: Experience) => this._onChange(experience)); } ngOnDestroy(): void { this._detroyed.next(); this._detroyed.complete(); } onTouched = () => {}; registerOnChange(fn: (experience: Experience) => void): void { this._onChange = fn; } registerOnTouched(fn: () => void): void { this.onTouched = fn; } writeValue(experience: Experience | undefined | null): void { this.experienceGroup.setValue(experience ?? EXPERIENCE_DEFAULT, { emitEvent: false, }); } setDisabledState(isDisabled: boolean): void { isDisabled ? this.experienceGroup.disable() : this.experienceGroup.enable(); } private _onChange = (experience: Experience) => {}; }
With this experience-form
component, we now have a standalone form section that generates an Experience
object on change and that can be understood as a simple FormControl
by Angular machinery.
Let’s embed it in global CV form!
Add experience-form into cv-form
If we wanted to add only one work experience by CV, the integration would be pretty easy:
<form [formGroup]="cvGroup" (submit)="addCv($event)"> <fieldset> <legend>Personal data</legend> <p> <label for="firstName">First name</label> <input type="text" id="firstName" formControlName="firstName" /> </p> <p> <label for="lastName">Last name</label> <input type="text" id="lastName" formControlName="lastName" /> </p> <p> <label for="birthDate">Date of birth</label> <input type="date" id="birthDate" formControlName="birthDate" /> </p> </fieldset> <experience-form [formControl]="experience"></experience-form> <div class="cv-form-actions"> <button type="button" (click)="addExperience()">ADD EXPERIENCE</button> <button type="submit">ADD CV</button> </div> </form>
@Component({ selector: 'cv-form', standalone: true, imports: [CommonModule, ReactiveFormsModule, ExperienceFormComponent], templateUrl: './cv-form.component.html', styleUrls: ['./cv-form.component.scss'], changeDetection: ChangeDetectionStrategy.OnPush, }) export class CvFormComponent { @Output() cvAdded = new EventEmitter<Cv>(); cvGroup = new FormGroup({ firstName: new FormControl('', { nonNullable: true, validators: Validators.required, }), lastName: new FormControl('', { nonNullable: true, validators: Validators.required, }), birthDate: new FormControl(new Date('2000-01-01'), { nonNullable: true, validators: Validators.required, }), experience: new FormControl(EXPERIENCE_DEFAULT), }); constructor() {} addCv(event: Event): void { event.preventDefault(); this.cvAdded.emit(this.cvGroup.value as Cv); this.cvGroup.reset(); } }
But here we would like a dynamic list of experience-form
added or removed by the applicant depending of his own work experiences. We will use here a very powerfull tool provided by Angular that is dedicated to this issue: FormArray
.
If you are not familiar with FormArray
, I suggest you this great article about its usage:
Angular FormArray - Complete Guide
In this post, you are going to learn everything that you need to know about the Angular FormArray construct, available…
With FormArray
we are able to add a dynamic array of FormControl
to our CV form and its value will be the array of every experience-form
value. Let’s do it!
cvGroup = new FormGroup({ ..., experiences: new FormArray([new FormControl(EXPERIENCE_DEFAULT)]), }); addExperience(): void { this.cvGroup.controls.experiences.push(new FormControl(EXPERIENCE_DEFAULT)); } removeExperience(index: number): void { this.cvGroup.controls.experiences.removeAt(index); }
<ul> <li class="cv-form-exp" *ngFor=" let expForm of cvGroup.controls.experiences.controls; let i = index " > <experience-form [formControl]="expForm"></experience-form> <button type="button" (click)="removeExperience(i)">X</button> </li> </ul>
Summary
We have just seen the power of ControlValueAccessor
to isolate parts of form and make them standalone components.
This practice enhances the single responsibility component principle and make the form sections reusable through an application or a multi applications project.
- 登录 发表评论